.NET MAUI is right around the corner and Preview 13 is out right now! There are so many great features packed into .NET MAUI and my favorite has to be all of the great Dependency Injection and configuration that goes into the MauiProgram startup. If you want to know more checkout my most recent YouTube video breaking down Preview 13 and all of the configuration that is built in including Essentials, Blazor, and more.
But, what if you want to do other things that aren't included out of the box... such as reading app configuration through a appsettings.json
file?!?!? Well you can, and it is super easy! Thanks to the MauiAppBuilder
we can use the ConfigurationManager
that is built in to configure settings in our .NET MAUI app. First things first, let's add the appsettings.json
file as an EmbeddedResource
:
{
"Settings": {
"KeyOne": 1,
"KeyTwo": true,
"KeyThree": {
"Message": "Oh, that's nice..."
}
}
}
Next, let's create a few classes that represent these configuration settings anywhere in the project:
public class Settings
{
public int KeyOne { get; set; }
public bool KeyTwo { get; set; }
public NestedSettings KeyThree { get; set; } = null!;
}
public class NestedSettings
{
public string Message { get; set; } = null!;
}
We will now need to configure our json
file with a few Microsoft.Extensions.Configuration
NuGet packages. Added the following into your .csproj
:
<ItemGroup>
<PackageReference Include="Microsoft.Extensions.Configuration.Binder" Version="6.0.0" />
<PackageReference Include="Microsoft.Extensions.Configuration.Json" Version="6.0.0" />
</ItemGroup>
Now, let's read in our json
file, and add it to our builder.Configuration
in our CreateMauiApp
method after we create the builder
:
var a = Assembly.GetExecutingAssembly();
using var stream = a.GetManifestResourceStream("MauiApp27.appsettings.json");
var config = new ConfigurationBuilder()
.AddJsonStream(stream)
.Build();
builder.Configuration.AddConfiguration(config);
The code above reads in the manifest resource from the assembly into a stream. You will want to change the MauiApp27
to whatever your assembly name is for your project. After that we load it up and add our new configuration to the builder's main ConfigurationManager
. We do this so we can dependency inject our IConfiguration
anywhere we want.
Note here that there will be some perf here for reading it in from the file. You should consider if you really need to use appsettings.json as your json is never going to change once compiled up.
We will want to add our MainPage
as a Transient into our IServiceCollection
in our CreateMauiApp
method:
builder.Services.AddTransient<MainPage>();
Now we can adjust our App.xaml.cs
to inject our MainPage:
public App(MainPage page)
{
InitializeComponent();
MainPage = page;
}
Then over on our MainPage
, we can inject our IConfiguration
and then use it when our button is clicked to read in our Settings and bind them together:
int count = 0;
IConfiguration configuration;
public MainPage(IConfiguration config)
{
InitializeComponent();
configuration = config;
}
private async void OnCounterClicked(object sender, EventArgs e)
{
count++;
CounterLabel.Text = $"Current count: {count}";
SemanticScreenReader.Announce(CounterLabel.Text);
var settings = configuration.GetRequiredSection("Settings").Get<Settings>();
await DisplayAlert("Config", $"{nameof(settings.KeyOne)}: {settings.KeyOne}" +
$"{nameof(settings.KeyTwo)}: {settings.KeyTwo}" +
$"{nameof(settings.KeyThree.Message)}: {settings.KeyThree.Message}", "OK");
}
Now, if you aren't using constructor injection don't worry as you can still access it manually by exposing the IServiceProvider
in our MauiProgram.cs
:
public static class MauiProgram
{
public static MauiApp CreateMauiApp()
{
//normal builder stuff
var app = builder.Build();
Services = app.Services;
return app;
}
public static IServiceProvider Services { get; private set; }
}
Then you can access it via: MauiProgram.Services.GetService();
Either way, you are good to go and you have access to these settings!!!
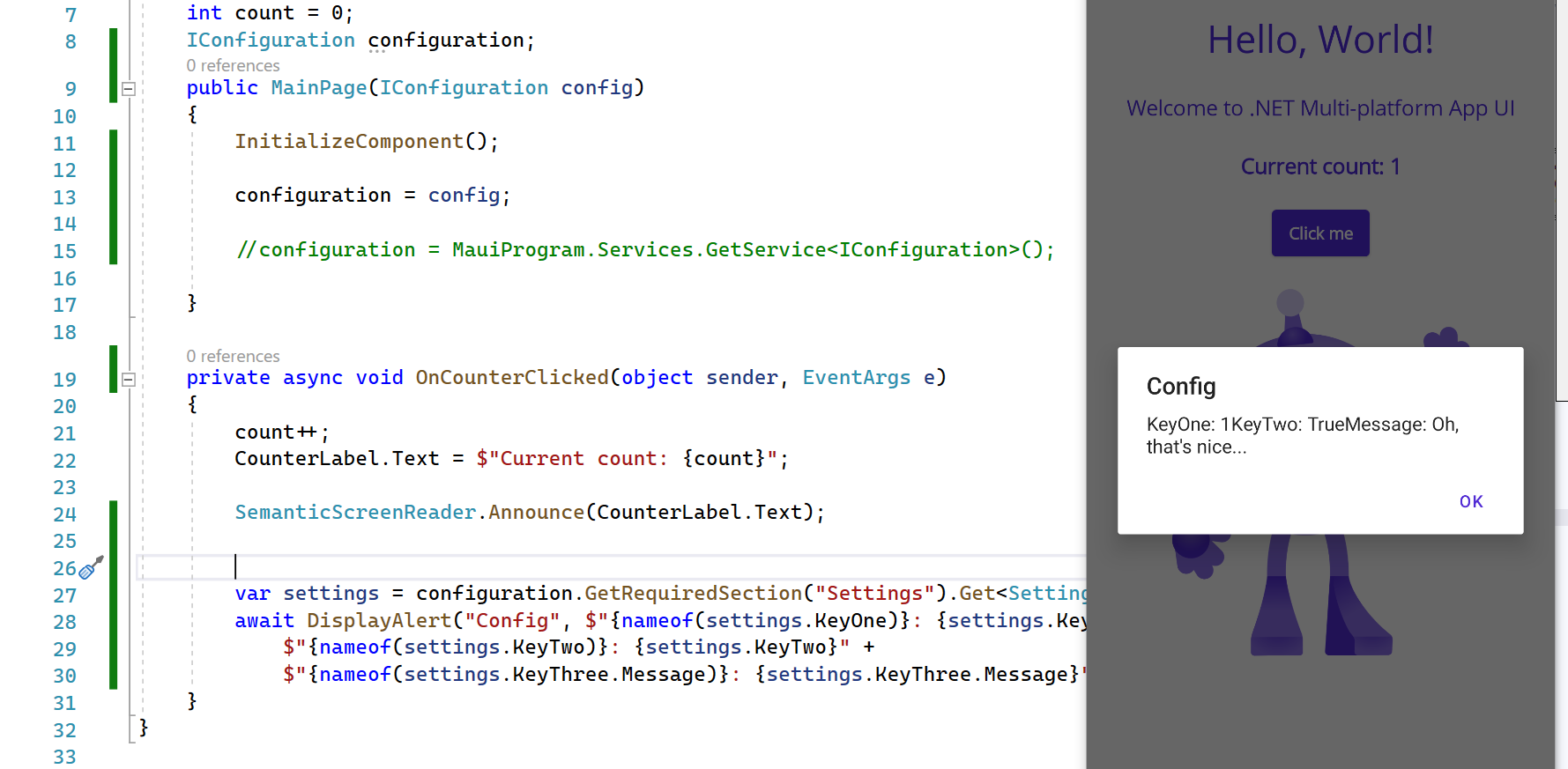
Want to learn more? Be sure to read the full documentation on Configuration in .NET. Full source code example can be found on GitHub right here!
Want to go farther? Check out Allan Ritchie's awesome Shiny Extensions library at https://github.com/shinyorg/configurationextensions
Checkout my videos on YouTube